

You also might want to render to texture, but I'll leave that as an exercise. This is a simple example, in reality you likely also want storage for the depth (and stencil) buffer. GlBindFramebuffer(GL_DRAW_FRAMEBUFFER,0) GlBindFramebuffer(GL_READ_FRAMEBUFFER,fbo) GlFramebufferRenderbuffer(GL_DRAW_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, GL_RENDERBUFFER, render_buf) GlBindFramebuffer(GL_DRAW_FRAMEBUFFER,fbo) GlRenderbufferStorage(GL_RENDERBUFFER, GL_BGRA8, width, height)
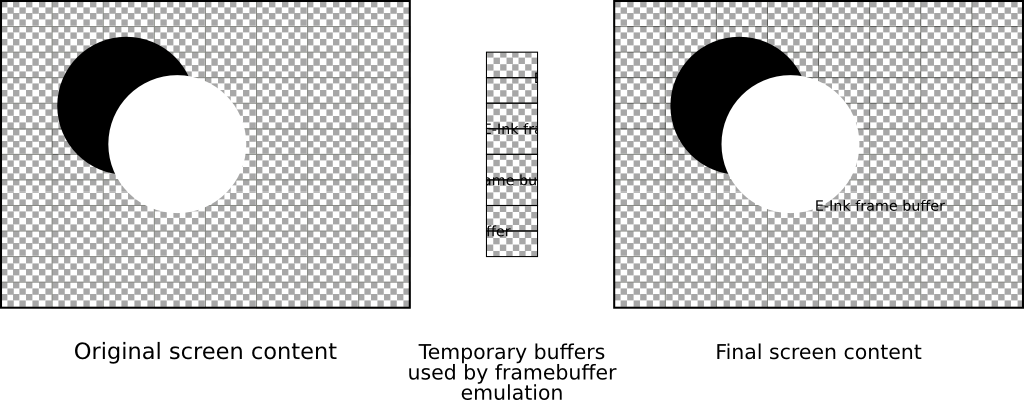
With this the code above would become something like this, again pseudo-code, so don't kill me if mistyped or forgot some statements. a naive "security camera" in a game), the latter if you just want to render/read-back. The first is optimal when you want to re-use the pixels in OpenGL itself as a texture (e.g. In practice, you can either draw to a texture or to a renderbuffer. That's where Framebuffer Objects come into play.Įssentially, an FBO lets you create a non-default framebuffer (like the FRONT and BACK buffers) that allow you to draw to a memory buffer instead of the screen buffers. Next to that, the front and back buffers are optimized to display pixels, not to read them back. We can emulate offscreen rendering by never swapping in the back buffer, but it doesn't feel right. We render to the screen buffers and read from those. First of all, we don't really do offscreen rendering do we. Technically you can also read the front buffer, but this is often discouraged as theoretically implementations were allowed to make some optimizations that might make your front buffer contain rubbish.

Note that you can also perfectly read the back buffer with the above method, clear it and draw something totally different before swapping it. You should call this before swapping the buffers. This will read the current back buffer (usually the buffer you're drawing to). GlReadPixels(0,0,width,height,GL_BGRA,GL_UNSIGNED_BYTE,&data) I use c++ pseudo code so it will likely contain errors, but should make the general flow clear: //Before swapping So a very basic offscreen rendering method would be something like the following. As is usual with OpenGL, the current buffer to read from is a state, which you can set with glReadBuffer. As you will notice in the documentation, there is no argument to choose which buffer. It all starts with glReadPixels, which you will use to transfer the pixels stored in a specific buffer on the GPU to the main memory (RAM).
